Spring Boot is a framework for building applications in Java. It makes it easy to create stand-alone and production-grade spring-based applications that you can just run. Spring was already there. Spring came because it reduces boilerplate code, but manual work and configuration are needed in a spring application.
The core spring framework already reduces boilerplate code and provides many helpful features for Java applications. Spring Boot especially focuses on reducing the effort required to set up and configure a spring application.
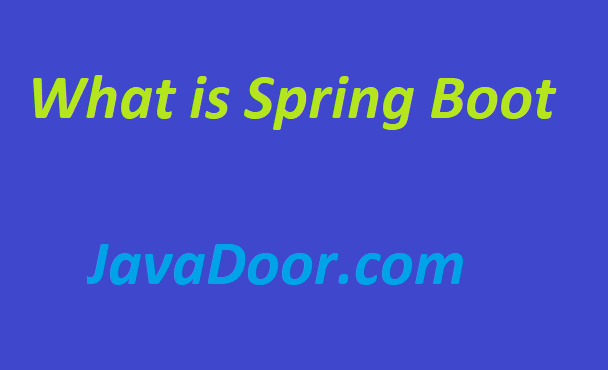
Previously we needed to have a server to deploy our application now the spring boot has an embedded server.
Without spring boot application-
POM.XML
<dependencies>
<dependency>
<groupId>org.glassfish.jersey.core</groupId>
<artifactId>jersey-server</artifactId>
<version>2.34</version>
</dependency>
<dependency>
<groupId>org.glassfish.jersey.containers</groupId>
<artifactId>jersey-container-servlet</artifactId>
<version>2.34</version>
</dependency>
</dependencies>
WEB.XML-
<web-app xmlns="http://java.sun.com/xml/ns/javaee" version="3.0">
<servlet>
<servlet-name>JerseyServlet</servlet-name>
<servlet-class>org.glassfish.jersey.servlet.ServletContainer</servlet-class>
<init-param>
<param-name>jersey.config.server.provider.packages</param-name>
<param-value>com.example.rest</param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>JerseyServlet</servlet-name>
<url-pattern>/api/*</url-pattern>
</servlet-mapping>
</web-app>
HelloResource.JAVA
package com.example.rest;
import jakarta.ws.rs.GET;
import jakarta.ws.rs.Path;
import jakarta.ws.rs.Produces;
import jakarta.ws.rs.core.MediaType;
@Path("/hello")
public class HelloResource {
@GET
@Produces(MediaType.TEXT_PLAIN)
public String sayHello() {
return "Hello, World!";
}
}
With Springboot application-
pom.xml
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
</dependencies>
HelloController.java
package com.example.demo;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class HelloController {
@GetMapping("/hello")
public String sayHello() {
return "Hello, World!";
}
}
DemoApplication.java
package com.example.demo;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class DemoApplication {
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
}
}
@SpringBootApplication annotation alone brings in a lot of preconfigured features, including component scan and embedded server configuration. which would take more time than traditional spring
Benefits of Spring Boot Application-
- Ease of Setup
- Embedded Servers
- Dependency Management
- Auto-Configuration
- Microservices Support
- Built-in Monitoring and Metrics
- Rapid Development
- Community and Ecosystem
- Reduced Boilerplate Code