In this post I will be post-interview questions about whatever I am facing in the interview so it will be easy for us to crack the interview, i have a total of 3.9 years of experience with Java, spring, and Spring Boot Microservices. It will be a mix of all the things.java interview questions and spring boot interviews.
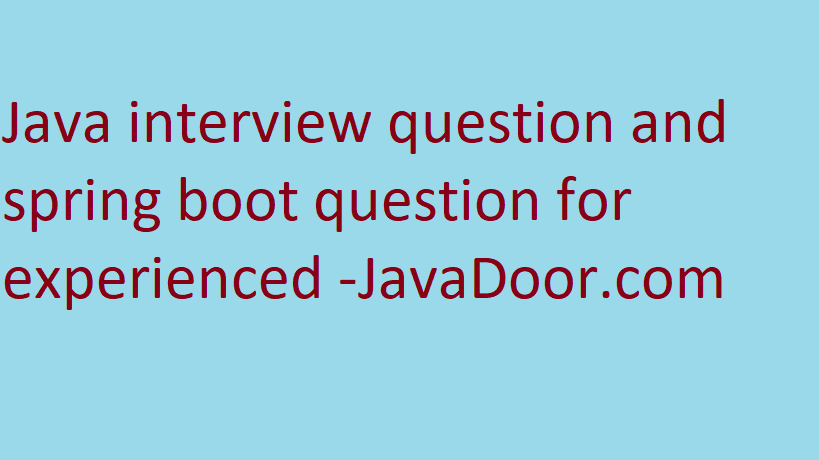
if it is a program I will write on my IDE and give you the best version of the code. and one more thing if you people have any suggestions for the question you can reply in the comments, and I will try to modify it.
Q-1. Anagram or not in the java8 program.
So basically there will be two strings we need to check if both strings contain the same character or not, my approach was first we need to sort both strings, and then we can check both strings.
import java.util.stream.Collectors;
import java.util.stream.Stream;
public class AnagramPractice {
public static void main(String[] args) {
String s1 = "ajay";
String s2 = "ayja";
boolean areAnagrams = isAnagram(s1, s2);
System.out.println("Are '" + s1 + "' and '" + s2 + "' anagrams? " + areAnagrams);
}
public static boolean isAnagram(String s1, String s2) {
if (s1 == null || s2 == null || s1.length() != s2.length()) {
return false;
}
String sortedS1 = s1.chars()
.sorted()
.mapToObj(c -> String.valueOf((char) c))
.collect(Collectors.joining());
String sortedS2 = s2.chars()
.sorted()
.mapToObj(c -> String.valueOf((char) c))
.collect(Collectors.joining());
return sortedS1.equals(sortedS2);
}
}
Q-2 Tell us about Spring boot cloud-config.
working on it
Q-3 What is serialization how do we implement
Serialization is a process where an object is converted into a byte stream so we can store a file, database, or transmitted over a network. The opposite process, converting stream back to object is called deserialization.
If a class wants to be serializable means the class should implement a serializable interface, this interface has no methods because it’s a marker interface –
use ObjectOutputStream for Serialization.
ObjectInputStream is used for DeSerialization.
Q4-Java CompletableFuture
is introduced in java8 to achieve asynchronous processes-
- Asynchronous Execution
- Chaining
- Combining Results
- Exception Handling
- Non-blocking
Run Synchronously-
CompletableFuture<String> futures = CompletableFuture.completedFuture("Java, Door");
System.out.println(futures.get()); // Prints: Java Door
Run Asynchronously-
CompletableFuture<Void> future = CompletableFuture.runAsync(() -> {
System.out.println("Running in a separate thread.");
});
Supply a Result Asynchronously:
CompletableFuture<String> future = CompletableFuture.supplyAsync(() -> {
return "Result from another thread.";
});
System.out.println(future.get()); // Prints: Result from another thread.
Chaining CompletableFuture
Using then apply:
Processes the result of the previous computation.
CompletableFuture<Integer> future = CompletableFuture.supplyAsync(() -> 5)
.thenApply(num -> num * 2);
System.out.println(future.get()); // Prints: 10
Using thenAccept:
Consumes the result without returning a value.
CompletableFuture.supplyAsync(() -> "Hello, Async!")
.thenAccept(result -> System.out.println(result));
Using the compose-
Chains tasks where the second task depends on the result of the first.
CompletableFuture<String> future = CompletableFuture.supplyAsync(() -> "Hello")
.thenCompose(result -> CompletableFuture.supplyAsync(() -> result + " World!"));
System.out.println(future.get()); // Prints: Hello World!
Combining Multiple CompletableFutures
Combines two futures’ results-
CompletableFuture<Integer> future1 = CompletableFuture.supplyAsync(() -> 10);
CompletableFuture<Integer> future2 = CompletableFuture.supplyAsync(() -> 20);
CompletableFuture<Integer> result = future1.thenCombine(future2, (a, b) -> a + b);
System.out.println(result.get()); // Prints: 30
Q-5: What is the inner class in Java and Example?
In Java, a class is defined inside a class that’s called the inner class. The benefit of the inner class is it accesses private members of the outer class They improve the readability and encapsulation of a class and will help include callbacks and event handling.
Q-6: Static (block, variable, method)–
Static variable-
- It will be available for all instances of a class.
- it belongs to a class rather than a specific instance.
- kit will loaded when the class is loaded.
class StaticVariable{
static int a=0; //static variable
}
Static block-
- Static block is also initialized when the class is loaded. It performs operations that need to be executed only once when the class is loaded.
Q-7: Stringbuffer and StringBuilder
StringBuilder and StringBuffer are classes in Java used for manipulating mutable sequences They have an initial capacity of 16 When the capacity exceeds, it grows dynamically.
it’s having different methods –
- append(String s) – Appends a string.
- reverse() – Reverses the string.
- insert(int offset, String s) – Inserts a string at the specified index.
- delete(int start, int end) – Deletes characters in a specified range.
- replace(int start, int end, String s) – Replaces characters in a range with the specified string.
- charAt(int index) – Returns the character at the specified index.
- capacity() and ensureCapacity(int minCapacity) – Get or ensure minimum capacity.
Criteria | StringBuffer | StringBuilder |
---|---|---|
Thread-Safety | Yes | No |
Performance | Slower | Faster |
Use Case | Multi-threaded apps | Single-threaded apps |
Default Capacity | 16 | 16 |
Synchronization | Synchronized | Not Synchronized in |